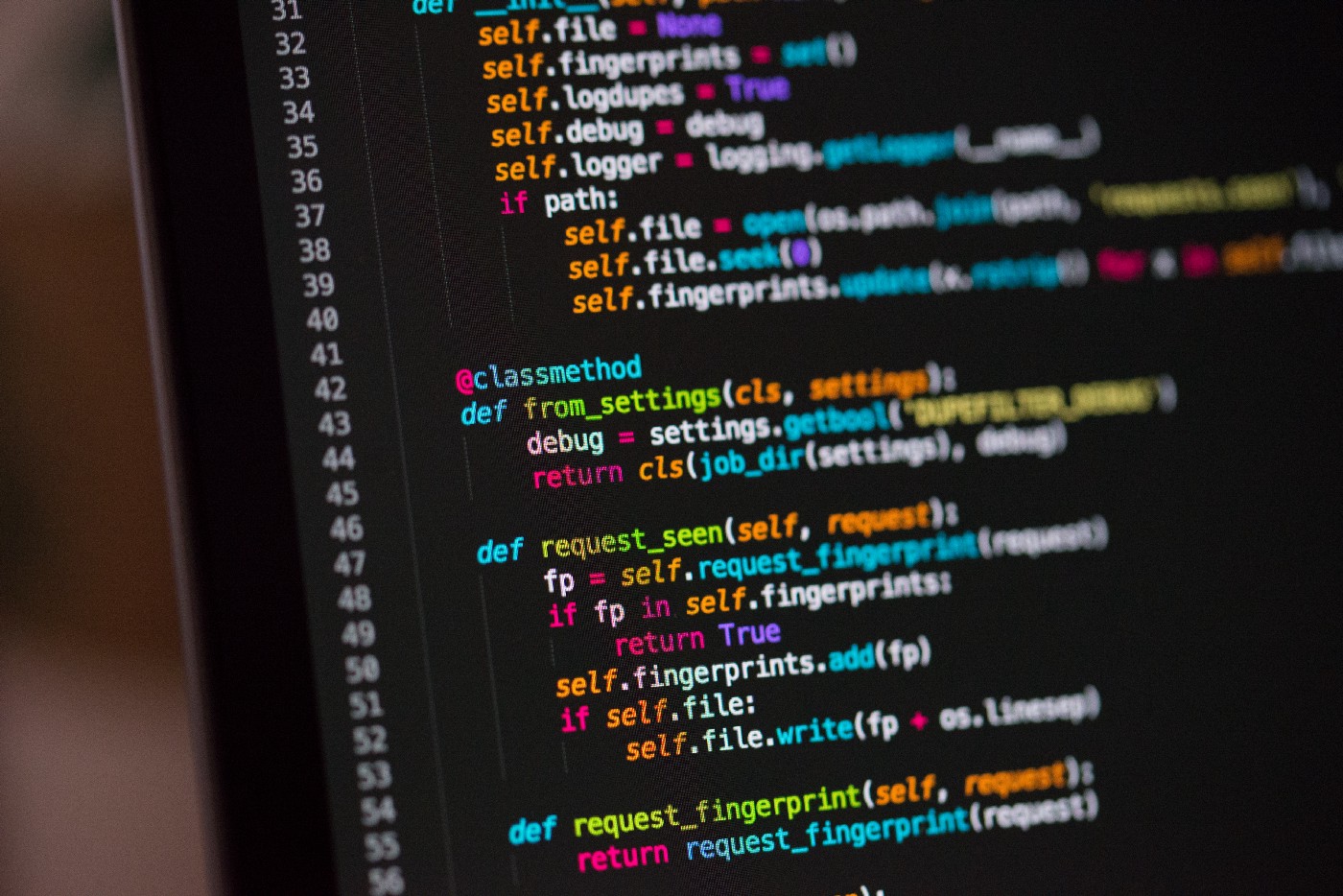
Node.js is an open-source JavaScript runtime environment that allows JavaScript code to be run and tested. It is an ideal tool for web development, creating websites, mobile applications, and cloud computing solutions.
It is fast, scalable, and efficient, and can be used in a wide range of applications. In this article, we will take a look at what Node.js is, its features, and some of the use cases where it can be used.
Since Node.js is considered a runtime environment, you may want to know what that means. In short, a runtime environment is a software program that runs code written in a specific programming language. The code is not directly executed by the computer's processor, but instead, it is interpreted by the runtime environment.
Features of Node JS
Node.js is a highly scalable platform that enables developers to create fast and efficient applications. It is built on top of the V8 JavaScript engine the JavaScript execution engine, which makes it very fast and was initially built for Google Chrome browser. Node.js also has a large ecosystem of open source libraries that can be used for various purposes.
Some of the features that make Node.js so popular are:
Asynchronous programming: Node.js uses an event-driven, non-blocking I/O model that makes it very efficient.
Highly Scalable: Node.js is very scalable thanks to its event-driven nature. It can handle a large number of concurrent connections with ease.
Cross-platform: Node.js can be used on any platform that supports JavaScript. This makes it very easy to develop applications that can be deployed on multiple platforms.
Rich ecosystem: There is a large ecosystem of open source libraries and tools available for Node.js, which makes it easy to develop and deploy applications.
Node.js has a large ecosystem of open source libraries and tools that make it easy to develop and deploy applications. These include libraries for various purposes such as logging, debugging, testing, and so on. There are also many tools available that can help you with task automation, monitoring, and performance tuning.
Several different unit testing frameworks are available for Node.js developers. A Node JS developer can create unit tests using Jest or Mocha and many others.
Node JS Development Teams
While, there is no exact number, but according to the Node.js Foundation, there are over 30,000 active Node.js developers and contributors worldwide. We think of that number as being on the lower side in terms of estimates. As Node's popularity drive more adoption and more developers to learn framework. The size of the ecosystem means it is easy to create a development team of node.js developers quickly.
Event Loop
In Node.js, JS code is executed on a single thread on an event basis - this is what's called an event loop. When Node.js processes a task, it does so on a single thread. This asynchronous processing can often lead to problems when Node.js receives a CPU bound task.
When this happens, Node.js will set aside all available CPU resources to process the task at hand, which can often result in slow processing and delays in the event loop.
Historically, this is why Node.js was not recommended for computationally heavy tasks. However, by utilizing tools such as web workers, it is possible to process these tasks in a more efficient manner. By doing so, it is possible to take advantage of the asynchronous processing model of Node.js while still avoiding the potential problems that can occur when processing CPU bound tasks.
Callback Function in Node JS
Node.js also makes use of a callback function to handle asynchronous tasks. Node.js developers can use the callback function call another function once a task has been completed. This allows Node.js to continue processing other tasks while the callback function is executed.
The use of an event loop and callback functions makes Node.js very efficient at handling asynchronous tasks. This is one of the reasons why it is so popular for developing software applications that require high levels of concurrency.
Node JS Libraries
Node.js uses several different libraries, including the JavaScript runtime engine V8, the libuv library, and the Node.js core library. These libraries work together to provide a fast and scalable platform for developing server-side applications.
V8 is a JavaScript runtime engine that is used by Node.js to execute code. It is fast and efficient, and provides all the features that are needed for running Node.js applications.
Node.js is built on top of the V8 JavaScript engine, but it also uses the libuv platform abstraction layer to provide cross-platform compatibility. The libuv platform abstraction layer is a library that provides support for asynchronous I/O. This means that it can handle tasks such as file I/O, network I/O, and process management without blocking the main thread of execution. This makes Node.js applications highly scalable and efficient.
The Node.js core library provides the functionality that is needed to build Node.js applications. It includes modules for working with the file system, network, process, and operating system.
Node Package Manager (NPM)
Node Package Manager is a package manager for Node.js that enables developers to share and reuse code. It also makes it easy to install and update Node.js libraries and tools. NPM is included in the Node.js distribution, so you don't need to install it separately. NPM is the largest ecosystem of open source libraries in the world.
NPM is a tool that enables developers to share and reuse code. It also makes it easy to install and update Node.js libraries and tools. NPM is included in the Node.js distribution, so you don't need to install it separately.
NPM is used to install and manage Node.js libraries and tools. You can use it to install a library or tool, and then include it in your Node.js application. NPM makes it easy to update libraries and tools, and you can use it to manage multiple versions of a library or tool.
Some benefits of using NPM include the ability to share code with other developers, ease of installation and updating, and the ability to manage multiple versions of a library or tool.
There are many useful NPM modules available, but some of the more popular libraries for Node.js developers include:
Express Framework
Express is a web application framework for Node.js that provides a range of features to help a Node JS developer develop web and mobile applications. It includes support for routing, handling requests and responses, and managing data. Express also makes it easy to develop applications that use the Model-View-Controller (MVC) pattern.
The benefits of using Express for Node.js developers include the following:
- Node.js developers can build faster and easier as Express provides a range of features out of the box
- It is lightweight and fast
- It is scalable and can be used for developing large applications
- And it can be easier to lear for a less senior node.js developer.
Socket.IO
Socket.IO is a library that enables real-time, bi-directional communication between clients and servers.
Mongoose
Mongoose is a MongoDB object modeling tool that helps you work with your data more efficiently. Mongoose is an object modeling tool that helps you work with your data more efficiently. It provides a layer of abstraction over MongoDB, making it easier to work with. Mongoose also has support for validation, query building, and more.
Some benefits of using Mongoose include simplifying your data model, providing built-in support for validation, and enabling you to work with your data more efficiently.
Webpack
Webpack is a module bundler that enables you to package your Node.js applications into static files that can be deployed on a web server. Webpack is a module bundler that enables you to package your Node.js applications into static files that can be deployed on a web server. This makes it easier to deploy and run your Node.js applications in a production environment.
Some benefits of using Webpack include the ability to deploy your Node.js applications to a static web server, and the ability to bundle your application into a single file.
Babel
Babel is a JavaScript compiler that enables you to use the latest JavaScript features in your applications. Babel enables you to use the latest JavaScript features in your applications. It also provides support for older browsers, so you can still use those features even if they are not supported by the browser. Babel is a JavaScript compiler that enables you to use the latest JavaScript features in your applications.
Some benefits of using Babel include the ability to use the latest JavaScript features, support for older browsers, and the ability to compile your code into a single file.
Async
Async is a utility module that provides various tools for working with asynchronous JavaScript. Async is a utility module that provides various tools for working with asynchronous JavaScript. Async provides a way to manage asynchronous code, such as code that requires a callback function. Async also provides features such as error handling and caching.
Some of the ways that async can be used include:
- Making sure that code is executed in the order that it is written
- Avoiding callback hell
- Error handling
- Cancelling asynchronous tasks
Async is a popular module because it makes working with asynchronous code more manageable. It is also easy to use, and provides a number of features that make it more powerful than other modules.
Passport
Passport is an authentication library that provides a simple and flexible way to authenticate users.
Great for Web Applications and other Node.js Use Cases
Node.js is suitable for developing a wide range of applications, such as:
- Web applications: Node.js can be used to develop both server-side and client-side applications.
- Network applications: Node.js can be used to develop network applications such as web servers, SSH servers, etc.
- Distributed systems: Node.js can be used to develop distributed systems.
- IoT applications: Node.js can be used to develop IoT applications.
- Microservices: Node.js is a popular platform for developing microservices.
- Command-line tools: Node.js can be used to develop powerful command-line tools.
Data Streaming Applications built using Node JS
Data Streaming Applications Industry giants, such as Netflix and Amazon Prime have heavily benefitted from NodeJS, as it ensures feather-light downloading and uploading speed for large amounts of data. With this, ads are flawlessly displayed, live TV shows are telecasted across mobile devices and official websites, and songs and movies are downloaded with ease.
Real-time chat applications and Chatbots use Node.js
Real-time chat applications: Have you ever wondered how Facebook Messenger or WhatsApp work? They use NodeJS for real-time communication between different users. The platform enables the server to push messages as soon as they are received, without any delay. Any kind of information can be exchanged including text, audio, and video.
Easier to Build APIs
API (Application Programming Interface) servers: API servers are built using NodeJS for smooth data retrieval from the client-side. These requests can be of any form- GET, POST, or DELETE. The platform also facilitates easy integration of third-party APIs.
Microservices Ready
Microservices: In this software development architecture, large applications are divided into individual services that work together. This makes the overall process much simpler and each service can be deployed independently. Also, since NodeJS is lightweight, it can be used to develop microservices without any hassle. Some benefits of microservices include: improved flexibility and scalability, easier to develop and deploy, reduced dependencies and improved fault tolerance.
NodeJS has become one of the most popular choices for enterprise web application development in a short span of time. It is being used by some of the biggest names in the industry, such as PayPal, Uber, Netflix, Trello, and eBay.
Netflix: Netflix is a popular streaming service that offers movies, TV shows, and documentaries.
LinkedIn: LinkedIn is a business-oriented social networking service.
eBay: eBay is an online marketplace where people can buy and sell items.
Uber: Uber is a ride-hailing service that allows people to request and pay for rides.
Trello: Trello is a project management application that helps users organize and track their work.
Node vs Other Backend Technologies for Web Development
Back-end development refers to the server-side of development. This involves the development of the back-end components of web applications, such as the database, application logic, and user management.
Some of the other backend technologies are:
- PHP: PHP is a general-purpose scripting language that is widely used for software development.
- Python: Python is a versatile language that can be used for application development, scientific computing, artificial intelligence, etc.
- Java: Java is a widely taught language that is used for developing enterprise applications.
- Ruby on Rails: Ruby on Rails is a popular web app development framework that is written in the Ruby.
When comparing Node.js to other backend technologies, it is important to consider the different features and use cases of each technology. In addition, Node.js applications are easy to maintain. The platform is constantly being updated with the latest security and performance fixes, so you don't have to worry about your application becoming outdated.
Node and Python
Node.js and Python are two of the most popular languages in the world. They are both widely used in software development, and they have many similarities. However, there are also some important differences between the two languages.
Node.js is based on JavaScript, whereas Python is a general-purpose language that can be used for a variety of tasks. Node.js is also asynchronous, meaning that it can execute multiple operations at the same time. This can make it more efficient than Python, which is synchronous.
However, Python is generally considered to be easier to learn than Node.js, so it may be a better choice for beginners. Ultimately, the best language for you will depend on your specific needs and preferences.
Python has a large standard library as well as multiple third-party libraries. It also supports multiple programming paradigms, such as object-oriented, procedural, and functional programming.
When comparing Node.js and Python, it is important to consider the areas where each excels. For example, Node.js is better suited for real-time pplications thanks to its event-driven architecture, while Python is more commonly used for data science and machine learning due to its extensive libraries. Ultimately, the best language to use depends on the specific needs of the project.
Node and C#
When it comes to coding languages, Node and C# both have a lot to offer. Both are widely used in the industry and have a strong community of developers. However, there are some key differences between the two languages.
Node is a javascript runtime that is built on Chrome's V8 JavaScript engine. It is used for developing server-side applications. On the other hand, C# is a multi-paradigm programming language developed by Microsoft. It is used for developing a range of applications, from web apps to desktop apps.
When it comes to syntax, Node uses an asynchronous programming model, which can be easier to learn for beginner programmers. C#, on the other hand, uses a synchronous programming model. This can make C# code more difficult to read and debug.
Node.js is often chosen for server-side applications that need to handle a lot of traffic, while C# is often chosen for desktop or mobile applications that require more user interface features.
However, both languages have their pros and cons and ultimately it comes down to personal preference. Node may be the better application development choice due to its easier syntax. However, if you're looking for more flexibility, C# may be a better choice. Whichever language you choose, there is a wealth of resources available online to help you get started.
One more thing. When it comes to web application development and developer opinion expect to get some very forceful answers why one is better than the other. Ultimately we view this as choice of preference, budget, and use case. In selecting the appropriate tools for your web applications or mobile app, keep in mind your ultimate business goals.
Node and Java
When Node.js came onto the scene, it promised to be a more lightweight and efficient alternative to Java. And while Node.js certainly has its advantages,Java is still a powerhouse when it comes to enterprise-grade development.
For one thing, Java is a statically typed language, which means that errors are caught at compile time rather than runtime. This can save a lot of headache down the line, as automated tests can catch potential bugs early on. Additionally, Java is backed by a large and active community of developers, which makes it easy to find support and resources when needed.
That said, Node.js has its own strengths that make it worth considering for certain projects. Its event-driven architecture makes it well-suited for developing real-time applications, such as chatbots or gaming servers. And since Node.js uses the JavaScript Runtime Environment, it can be a good choice for projects where front-end and back-end web development are being done by the same development teams.
In the end, the decision of whether to use Node.js or Java comes down to what kinds of features and capabilities are most important for the project at hand.
PHP and Node.
PHP is a programming language that has been around for many years. Over the course of its history, it has developed a reputation for being difficult to work with and inefficient. As a result, many developers have turned to other languages, such as Ruby on Rails or Node.js.
However, PHP remains popular among some circles, particularly in the world of web development. There are a number of reasons why developers might still choose to use PHP, despite its drawbacks.
It is relatively easy to learn, and there is a large amount of online documentation available. PHP integrates well with other technologies, such as HTML and CSS. And its long history translates into it powering some of the world's most popular websites, including Facebook and just about everything related to WordPress.
Since it has been around for more than two decades, it has also gained a reputation for being chaotic and unpredictable. As a result, many developers have come to view PHP with suspicion and even hate.
There are several reasons for this attitude. The PHP ecosystem while large is poorly managed and unfocused. This leads to constant changes within PHP, with new features and syntax being added on a regular basis. Â So you get a plethora of competing frameworks and tools. This can make it difficult to keep up.
PHP code is often messy and "hacky," making it hard to read and debug. And PHP's built-in functions are often inconsistent, which can lead to confusion and errors.
In our view, most PHP written applications will ultimately be migrated to new frameworks and languages over time as developers sour on PHP.
Goals of Node
One of Node.js' strongest goals is to provide scalable network applications and change the way connections are made to the web server.
Node makes the use of Event-Driven programming approach Instead of spawning a new OS thread for each network connection and allocating the required memory for it (just like the way Java, PHP works).
Each connection fires an event run within the Node's engine's process. Since there are no locks, it becomes very easy to have multiple processes running in parallel on a single thread. This is one of the main reasons why Node scales so well.
Node and Mobile App Development
There are several reasons why NodeJS mobile app development is a good idea for 2021. First, NodeJS can create real-time Web apps that process a high volume of short messages with minimal delay. This is ideal for mobile app development where users expect near-instant responses. For example, Yahoo, LinkedIn, and Uber are built on NodeJS.
Since NodeJS is lightweight and efficient, it is well-suited for mobile app development. It can run on devices with limited resources, such as smartphones, without compromising performance.
Scalability is an actual strength of NodeJS because it allows building apps that can handle a large number of users without any issue. This is due to the fact that NodeJS uses a non-blocking I/O model which makes it very efficient in handling concurrent requests.
How to Hire Node JS Developers
Node JS developers are in high demand. This is due to the popularity of Node.js. If you are looking to hire Node JS developers, there are a few things you should keep in mind. Hire Node JS developers with experience working on the service you are trying to build. Given the range of applications node.js developers can build simply having an understanding of Node is no long enough. Â
Depending on the stack you are building try to make sure they have the requisite stack in the background. Â For instance, Node.js developers who are familiar with the MEAN stack (MongoDB, Express.js, AngularJS, and Node.js) may be perfect for one project but not if you are looking for full-stack Node JS programmers with React skills.
At Azumo, our approach to hire node js developers is to look for well rounded individual contributors, leaders and architects. Â So each js developer we hire has the ability to fit into our portfolio of js projects.
Is a Node.js Developer Budget Friendly?
Finding a Node.js developer can be expensive and it is important to know what you are looking for when hiring one. It is possible to find Node.js developers on a budget by looking in the right places.
At Azumo, we focus on hiring Node.js developers. We have a strong understanding of the market and what to look for when hiring a Node js developer as web developer vs a node js developer with skills using a microservices architecture.
Our Node JS experts can focus on designing the best architecture and choosing new technologies that can speed the development process or create scalable applications. Â Our approach goes beyond how to hire a node.js developer or the hourly rates. Our extensive experience enables to us to deliver on your project with a dedicated team fit for your needs.
JavaScript Everywhere
Node.js completely changes the way we think about JavaScript. It allows us to write code that runs on the server side, which was once the domain of PHP, Java, and other languages.
But Node.js is more than just a server-side scripting language. It also includes a rich set of libraries and tools that allow us to build full-fledged applications. This makes Node.js a powerful platform for enterprise-grade development.
In the past, JavaScript was primarily used for client-side development, in the form of web browsers. But with Node JS, JavaScript can now be used for server-side development as well. This makes it possible to write code that runs on the server and the client, making for a more seamless experience for developers.
Hire the best Node developers from Azumo!